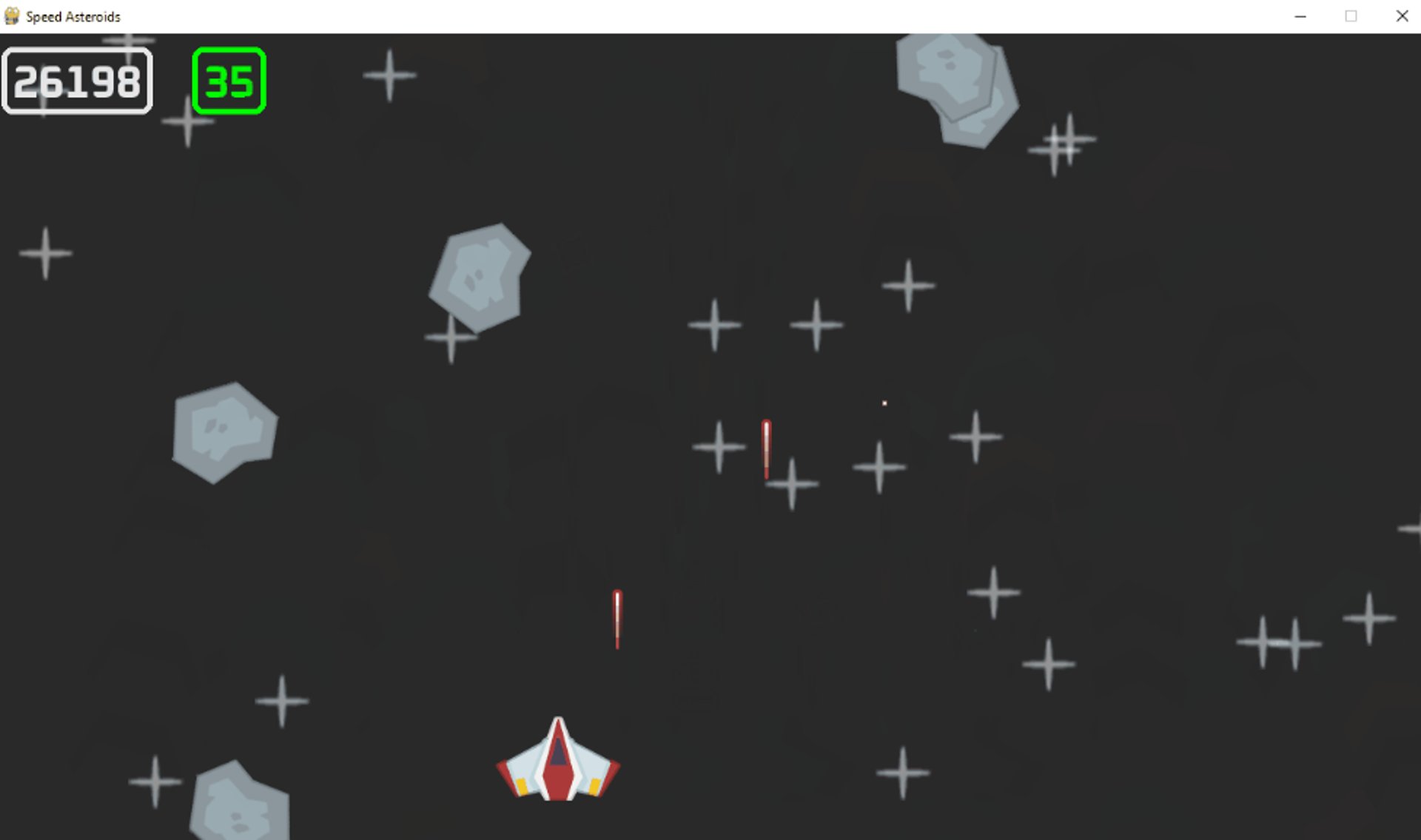
Python OOP Hands-on Practice: Development of a Space Shooter Game
Python Pygame Game Development with OOP principles
Tech Stack
Python 3, OOP, pygame-ce
VsCode, Jupyter, GitHub
Project
Development of a pygame Space Shooter game using the Python OOP principles, code structure and basic game design principles
Benefits
Object Oriented Programming helps Data specialists write cleaner, more maintainable, and scalable analysis pipelines. Building code on a robust structure with the help of OOP principles, Python loops and its modular capabilities is fundamental.
Goals
Enhancing my Python coding and game development skills.
Find all the code of the game in this Github repo. Want to play the game? Download the game file and install it (Windows only).
You can find the first OOP project here (simple text-based combat game).
As a junior data analyst deepening my Python skills and aspiring to transition into data science and machine learning engineering, I’ve recently explored object-oriented programming (OOP) more thoroughly through a hands-on project: building a retro shooter game with Pygame. This article outlines the core Python principles I applied, ranked by importance to the success and structure of the game, and how they translate into practices I find increasingly valuable in data analysis workflows — particularly for structuring robust, maintainable pipelines and modular analytics tools.
Object-Oriented Programming (OOP)
Essential – The entire game is structured around OOP.
Pygame's architecture naturally encourages object design. Each entity in the game (player, enemies, projectiles, UI elements) is modeled as a class that inherits from pygame.sprite.Sprite:
This design principle directly translates to data analysis workflows. For example, reusable components like DataCleaner, Preprocessor, or ChartBuilder can be modeled as classes. This approach allows each class to encapsulate logic and state, improving readability and reusability in ETL pipelines or notebook-driven analysis.
Encapsulation
Essential – Keeps game logic modular and maintainable.
Each sprite class encapsulates its own behavior (update, move, collide, etc.). This separation of concerns is vital in reducing side effects and increasing testability:
In data analysis, encapsulating transformation logic within discrete functions or classes avoids entangling multiple steps of a workflow. It's a great exercise in keeping logic traceable, a skill that's critical when debugging long notebooks or building dashboards.
Inheritance
Highly important – Enables polymorphism across game entities.
All entities extend pygame.sprite.Sprite, gaining common functionality such as group updates, drawing, and collision detection:
In data workflows, inheritance is useful when extending base classes for plotting utilities or data loaders. In ML, custom classes can inherit from base interfaces for cross-validation strategies or model wrappers. Learning this pattern in a game helps cement its usefulness in more analytical contexts.
Modular Imports and Path Handling
Important – Manages cross-file dependencies cleanly.
Using a utils-pyg folder for reusable functions and defining asset_path() to resolve resource paths ensures portability:
Event-Driven Programming
Important – Models real-time input and user interaction.
The pygame.event.get() loop listens for keyboard and timer-based events, which echoes how dashboards or interactive tools respond to user events:
Although less common in standard data scripts, this type of structure is directly relevant to web dashboards (e.g., Plotly Dash or Streamlit) and asynchronous data listeners.
Procedural Logic for Setup
Supportive – Handles initialization and layout.
While most of the logic is class-based, game setup is handled procedurally: asset loading, screen setup, and event registration. Similarly, in data work, setup scripts define environment variables, read credentials, or configure visualization themes:
Why Pygame is Great Practice for Python Developers in Data Fields
1. Forces you to think in objects
You learn how to model behaviors and data attributes through objects — just like building reusable ETL or feature engineering components.
2. Gives immediate visual feedback
You can directly see how changes in logic affect behavior — a great way to understand side effects and debugging.
3. Reinforces code modularity
Separating game entities helps mirror clean analytics pipelines, where tasks are split into focused stages.
4. Encourages performance awareness
Delta-time tracking and sprite batch updates are like optimizing memory in pandas or reducing redundant joins in SQL:
Final Thoughts
Building this game didn’t just strengthen my Python fundamentals — it mirrored real-world engineering patterns I aim to master. Clean abstractions, modular design, and object-centric logic will support me as I grow toward data science and ML engineering roles.
If you're a junior analyst or new Python learner, consider building a small game like this. It’s a practical, playful way to apply serious programming concepts — all while learning how to write maintainable, production-level Python code.
💡 If you want to explore other topics like SQL, check my full SQLAlchemy journey in my GitHub repository.